닉네임을 설정한 후 로비에 입장한다.
public override void OnJoinedLobby()//로비에 접속하면??
{
PhotonNetwork.LocalPlayer.NickName = NickNameInput.text;//포톤 로컬 플레이어 닉네임을 설정
NickName.text = PhotonNetwork.LocalPlayer.NickName;//닉네임 text를 로컬 플레이어 닉네임으로 설정
print(PhotonNetwork.LocalPlayer.NickName);
LoginPanel.SetActive(false);
StartPanel.SetActive(true);
myList.Clear();
}
OnJoinedLobby 함수는 로비에 접속할 때 실행된다. 이 함수를 재정의하여 동작을 변경할 수 있다.
방이 존재하지 않을 때 바로 시작을 누르면 오류 문구를 띄운다.
public override void OnJoinRandomFailed(short returnCode, string message)
{
방없음Panel.SetActive(true);
print("방랜덤참가실패");
}
한 플레이어가 방을 생성한다.
public void CreateRoom() => PhotonNetwork.CreateRoom(roomInput.text, new RoomOptions { MaxPlayers = 8 });
확인 버튼을 누르면 CreateRoom() 함수를 호출한다.
public override void OnJoinedRoom()
{
RoomName.text = PhotonNetwork.CurrentRoom.Name;
RoomPanel.SetActive(true);
StartPanel.SetActive(false);
MakeRoomPanel.SetActive(false);
print("방참가완료");
}
제대로 방이 만들어 졌다면 OnJoinedRoom() 함수를 호출한다.
public override void OnRoomListUpdate(List<RoomInfo> roomList)
{
int roomCount = roomList.Count;
for (int i = 0; i < roomCount; i++)
{
if (!roomList[i].RemovedFromList) //만약 방이 제거되지 않았다면??
{
if (!myList.Contains(roomList[i])) myList.Add(roomList[i]);//myList에 없다면?? 추가한다.
else myList[myList.IndexOf(roomList[i])] = roomList[i];//myList에 이미 있다면?? update한다.
}
else if (myList.IndexOf(roomList[i]) != -1) myList.RemoveAt(myList.IndexOf(roomList[i]));//제거 될 방인데 만약 mylist에 제거할 방이 있다면?? 제거해라.
/*else
{
myList.Remove(roomList[i]);
}*/
}
MyListRenewal();
}
방이 생성되면 새로운 RoomList를 업데이트해야 한다. 이 과정에서 받는 roomlist는 최신 상태의 목록이다.
void MyListRenewal()
{
// 최대페이지
maxPage = (myList.Count % CellBtn.Length == 0) ? myList.Count / CellBtn.Length : myList.Count / CellBtn.Length + 1;//만약 myList.Count가 3이라면?? (3%6) maxpage는 1(0+1)이 된다.
// 이전, 다음버튼
PreviousBtn.interactable = (currentPage <= 1) ? false : true;//현재 page가 1이라면?? PreviousBtn을 활성화할 필요는 없다. 현재 페이지가 2 이상일 때 활성화
NextBtn.interactable = (currentPage >= maxPage) ? false : true;//현재 page가 maxpage보다 작다면 NextBtn을 활성화. maxPage가 3까지 차있고 현재 page가 1or2 이라면 활성화.
// 페이지에 맞는 리스트 대입
multiple = (currentPage - 1) * CellBtn.Length;//page에 맞는 방을 보여주기 위해 multiple을 사용
for (int i = 0; i < CellBtn.Length; i++)
{
CellBtn[i].interactable = (multiple + i < myList.Count) ? true : false;//현재 존재하는 방일 경우?? myList.Count가 2이라면 0,1번째 CellBtn은 활성화 되고 2,3,4,5번째 CellBtn은 비활성화 된다.
CellBtn[i].transform.GetChild(0).GetComponent<Text>().text = (multiple + i < myList.Count) ? myList[multiple + i].Name : "";
CellBtn[i].transform.GetChild(1).GetComponent<Text>().text = (multiple + i < myList.Count) ? myList[multiple + i].PlayerCount + "/" + myList[multiple + i].MaxPlayers : "";
CellBtn[i].transform.GetChild(2).GetComponent<Text>().text = (multiple + i < myList.Count) ? (multiple+i).ToString() : " ";
if (multiple + i < myList.Count)
{
CellBtn[i].transform.GetChild(3).GetComponent<Image>().gameObject.SetActive(true);
}
else
{
CellBtn[i].transform.GetChild(3).GetComponent<Image>().gameObject.SetActive(false);
}
}
}
MyListRenewal()에서는 방 목록을 활성화할지 비활성화할지 결정한다.
대기실에 방이 표시된다.
방에 들어가면 "??님이 참가했습니다"라는 메시지가 나타난다.
자신의 채팅은 노란색으로 표시되도록 설정해두었다.
채팅 구현하는 부분이 조금 헷갈렷는데
옆에 위치한 나팔 아이콘을 누르면 Send() 함수가 호출된다.
public void Send()
{
string senderName = PhotonNetwork.NickName;
PV.RPC("ChatRPC", RpcTarget.All, PhotonNetwork.NickName + " : " + ChatInput.text, senderName);
ChatInput.text = "";
}
이 함수는 모든 플레이어에게 ChatRPC 함수를 호출하여 채팅 메시지를 전달한다.
void ChatRPC(string msg, string senderName)
{
if (senderName == PhotonNetwork.NickName)
{
msg = "<color=yellow>" + msg + "</color>";
}
else
{
msg = "<color=black>" + msg + "</color>";
}
bool isInput = false;
for (int i = 0; i < ChatText.Length; i++)
if (ChatText[i].text == "")
{
isInput = true;
ChatText[i].text = msg;
break;
}
if (!isInput) // 꽉차면 한칸씩 위로 올림
{
for (int i = 1; i < ChatText.Length; i++) ChatText[i - 1].text = ChatText[i].text;
ChatText[ChatText.Length - 1].text = msg;
}
}
보낸 사람이 본인일 경우, 채팅 메시지는 노란색으로 표시되며, 그렇지 않은 경우에는 검정색으로 표시된다.(흰색이 좋을 듯)
public override void OnPlayerEnteredRoom(Player newPlayer)
{
ChatRPC("<color=yellow>" + newPlayer.NickName + "님이 참가하셨습니다</color>", newPlayer.NickName);
}
public override void OnPlayerLeftRoom(Player otherPlayer)
{
ChatRPC("<color=yellow>" + otherPlayer.NickName + "님이 퇴장하셨습니다</color>", otherPlayer.NickName);
}
또한, 누군가 방에 들어오거나 나갈 때마다 ChatRPC가 호출되도록 설정하였다.
public override void OnLeftRoom()
{
for (int i = 0; i < ChatText.Length; i++)
{
ChatText[i].text = "";
}
PhotonNetwork.JoinLobby();
RoomPanel.SetActive(false);
StartPanel.SetActive(true);
}
스크롤이 자동으로 내려가도록 구현했지만, 현재 정상적으로 작동하지 않는다. 추후 수정이 필요하다.
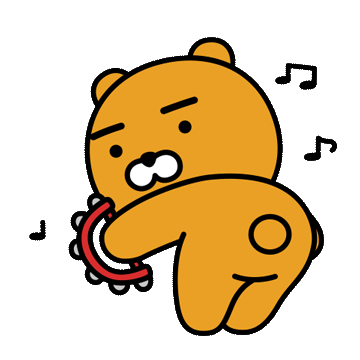
'UNITY > 크레이지아케이드 모작' 카테고리의 다른 글
캐릭터 움직임 구현 (0) | 2024.07.18 |
---|---|
방 구현(Ready) (0) | 2024.07.16 |
오류 모음 (0) | 2024.07.12 |
크아 모작 기본적인 버튼 구현 (0) | 2024.06.28 |
크아 모작 프로젝트 시작 (1) | 2024.06.26 |